While learning Windows Phone development you might want to try your skills in creating some cool apps. Most probably some of those apps requires you to create multiple pages in your application, which needs you to have some idea about navigation between multiple pages and passing data between them.
Let's start with a new "Blank App (Windows Phone)" project and name it as "NavigationDemo". We'll get MainPage.xaml as the default page already added in the solution. Now let's add another "Blank Page" called Page1.xaml.
Now we will try to navigate from MainPage.xaml to Page1.xaml. Open MainPage.xaml page and replace the Grid with following xaml code.
<StackPanel Margin="40">
<TextBlock Text="Main Page" FontSize="25"/>
<Button Content="Go To Page 1" Click="Button_Click"/>
</StackPanel>
We have just added the UI elements to help us navigate to Page1, but still we haven't added the actual code for implementing navigation. Just right click on button click event name and select "Go To Definition" and it will take you to the code behind file MainPage.xaml.cs with a new event handler method with event name.
Now add the following code inside button event handler.
// Navigating to Page 1
Frame.Navigate(typeof(Page1));
and our button event handler will look like as follows.
private void Button_Click(object sender, RoutedEventArgs e)
{
// Navigating to Page 1
Frame.Navigate(typeof(Page1));
}
At this moment if you'll run your application and press "Go To Page 1" button in MainPage.xaml, you will be redirected to Page1 having a blank screen as we haven't yet added anything visible in Page1.xaml. Now let's add another "Blank Page" and give it name as Page2.xaml. This time while navigating from Page1.xaml to Page2.xaml, we will also pass data in form of parameter. Before that we need to make following changes in Page1.xaml file.
Let's replace Grid with following xaml code in Page1.xaml file.
<StackPanel Margin="40">
<TextBlock Text="Page 1" FontSize="25"/>
<Button Content="Go To Page 2 With Data" Click="Button_Click"/>
</StackPanel>
Now right click on button click event name and select "Go To Definition" which will take you to the code behind file Page1.xaml.cs with a new event handler method with event name. Add the following code inside button event handler.
Frame.Navigate(typeof(Page2), "Sample data from Page1");
and our button event handler will look like
private void Button_Click(object sender, RoutedEventArgs e)
{
// Navigating to Page2 with parameter
Frame.Navigate(typeof(Page2), "Sample data from Page1");
}
So far we have added functionality to navigate and pass data from page Page1.xaml to Page2.xaml. Now lets open Page2.xaml page and add a TextBlock in UI to show the parameter that we are going to receive from Page1.xaml.
Let's replace Grid with following xaml code in Page2.xaml file.
<StackPanel Margin="40">
<TextBlock Text="Page 2" FontSize="25"/>
<TextBlock x:Name="tblShowParameter" FontSize="25"/>
<Button Content="Go Back" Click="Button_Click"/>
</StackPanel>
We'll retrieve passed value using OnNavigatedTo event that should already be generated in code behind file of Page2.xaml.cs. The default definition for OnNavigatedTo event is as follows.
/// <summary>
/// Invoked when this page is about to be displayed in a Frame.
/// </summary>
/// <param name="e">Event data that describes how this page was reached.
/// This parameter is typically used to configure the page.</param>
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
As mentioned in the auto-generated comment this Page event is invoked when Page is about to be displayed in a Frame. Here parameter "NavigationEventArgs" is used to fetch the value passed from calling page. Let's add the following code inside this Page event to retrieve the passed value and show it in the TextBlock tblShowParameter.
tblShowParameter.Text = e.Parameter.ToString();
In the preceding xaml code for Page2.xaml, you might notice that we have also introduced a "Go Back" button. We'll see Frame object to be useful for this purpose also. The Frame actually keeps track of the pages and also maintains the order in which they are visited. To implement "Go Back" button in page Page2.xaml, first switch to design mode and right click on button click event name to select "Go To Definition". Add the following code inside the generated event.
Frame.GoBack();
Once you'll start running this application from the beginning, you'll see the navigation flow as depicted in the following image.
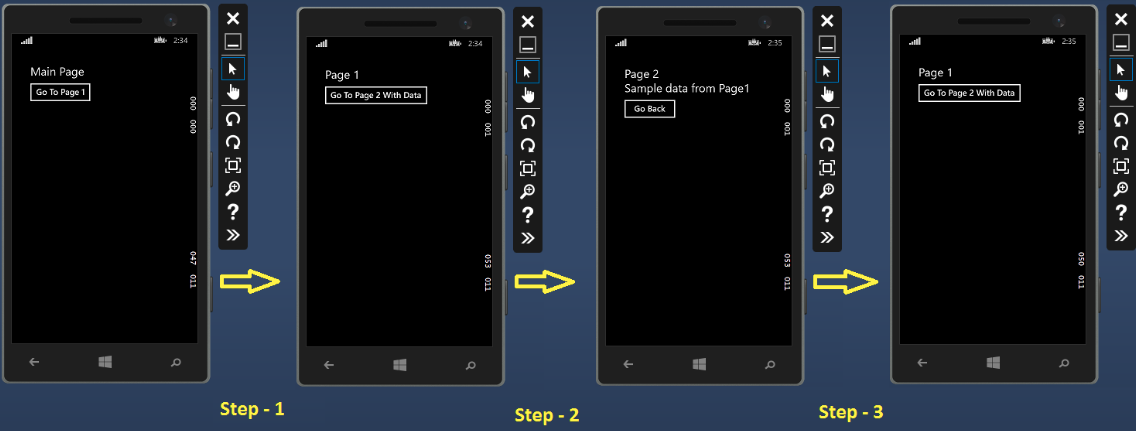
Here Step-1 shows navigation flow from MainPage.xaml to Page1.xaml, Step-2 shows navigation flow from Page1.xaml to Page2.xaml and Step-3 shows navigation flow from Page2.xaml to Page1.xaml using "Go Back" button.
0 Comment(s)