Job Scheduling Api
If your app used long repetitive task, you must be using activities and mostly the services and timer tasks but sometimes system stops the services and activities to free up memory and space.
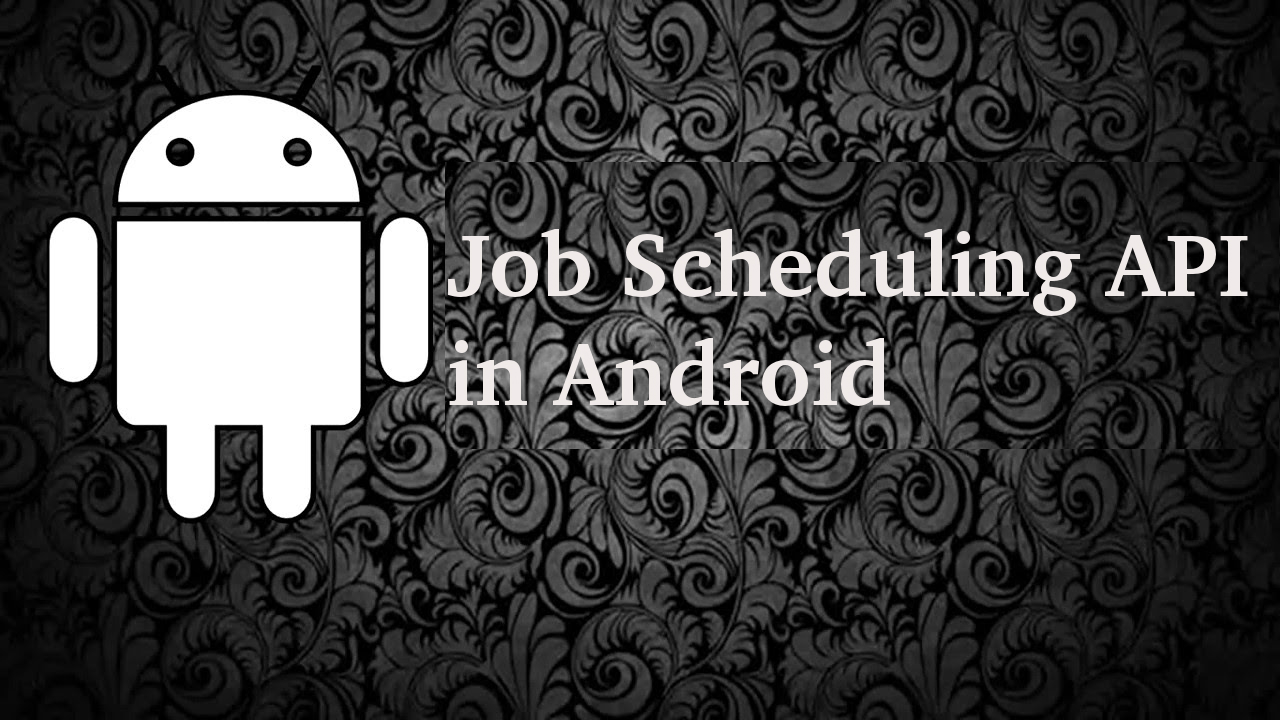
Android system currently uses Alarm Manager and JobSchedular to achieve the repetitive task, where JobSchedular is the latest one and pretty useful.From Android 5.0(Lollipop) added the Job scheduling API.
ADVANTAGES
JobScheduler API has a process of batch scheduling of jobs. Battery consumption can be reduced by combining the jobs.Uploads are easier using JobManager. Job scheduler also handles when the application restarts.
Read the following when should we can use this API.
JobInfo
Jobinfo object specifies the scheduling functionality The job scheduler allows to consider the state of the device, You can use the JobInfo.Builder class to allow and configure schedule task to run.
Note the following points for scheduling task under some conditions:
For implementing Job, you should user JobService class and implement the onStartJob and onStopJob methods. onStartJob is performed on the UI thread.
JobService should be registered in the Android manifest with the BIND_JOB_SERVICE permission.
<service
android:name=".DemoJobService"
android:label="Demo service"
android:permission="android.permission.BIND_JOB_SERVICE" >
</service>
Create Job
**
* JobService to be scheduled by the JobScheduler.
* start another service
*/
public class DemoJobService extends JobService {
private static final String TAG = "DemoService.class.getName";
//The onStartJob is performed in the main thread, if you start asynchronous processing in this
//method, return true otherwise false.
@Override
public boolean onStartJob(JobParameters params) {
Intent service = new Intent(getApplicationContext(), LocalService.class);
getApplicationContext().startService(service);
Util.scheduleJob(getApplicationContext()); // reschedule the job
return true;
}
//If the job fails for some reason, return true from on the onStopJob to restart the job.
@Override
public boolean onStopJob(JobParameters params) {
return true;
}
}
Add Receiver Class
Register receiver android.intent.action.BOOT_COMPLETED
broadcast and Schedule the Job
/*Broadcast receiver
*
*/
public class TestServiceReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
//event fire when boot is completed
Utility.scheduleJob(context);
}
}
//Register the receiver in the Android manifest
<receiver android:name="TestServiceReceiver" >
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED" />
</intent-filter>
</receiver>
public class Utility {
// scheduling start of the Jobservice every 10 - 20 secs
public static void scheduleJob(Context context) {
ComponentName serviceComponent = new ComponentName(context, DemoJobService.class);
//create and schedule a jobinfo builder
JobInfo.Builder builder = new JobInfo.Builder(0, serviceComponent);
builder.setMinimumLatency(1 * 1000); // waiting time
builder.setOverrideDeadline(20 * 1000); // max delay
// device idle true
builder.setRequiresDeviceIdle(true);
// works even on charging and discharging
builder.setRequiresCharging(false);
JobScheduler jobScheduler = context.getSystemService(JobScheduler.class);
jobScheduler.schedule(builder.build());
}
}
0 Comment(s)